Circular reference detected in Flutter: Causes and How to Fix
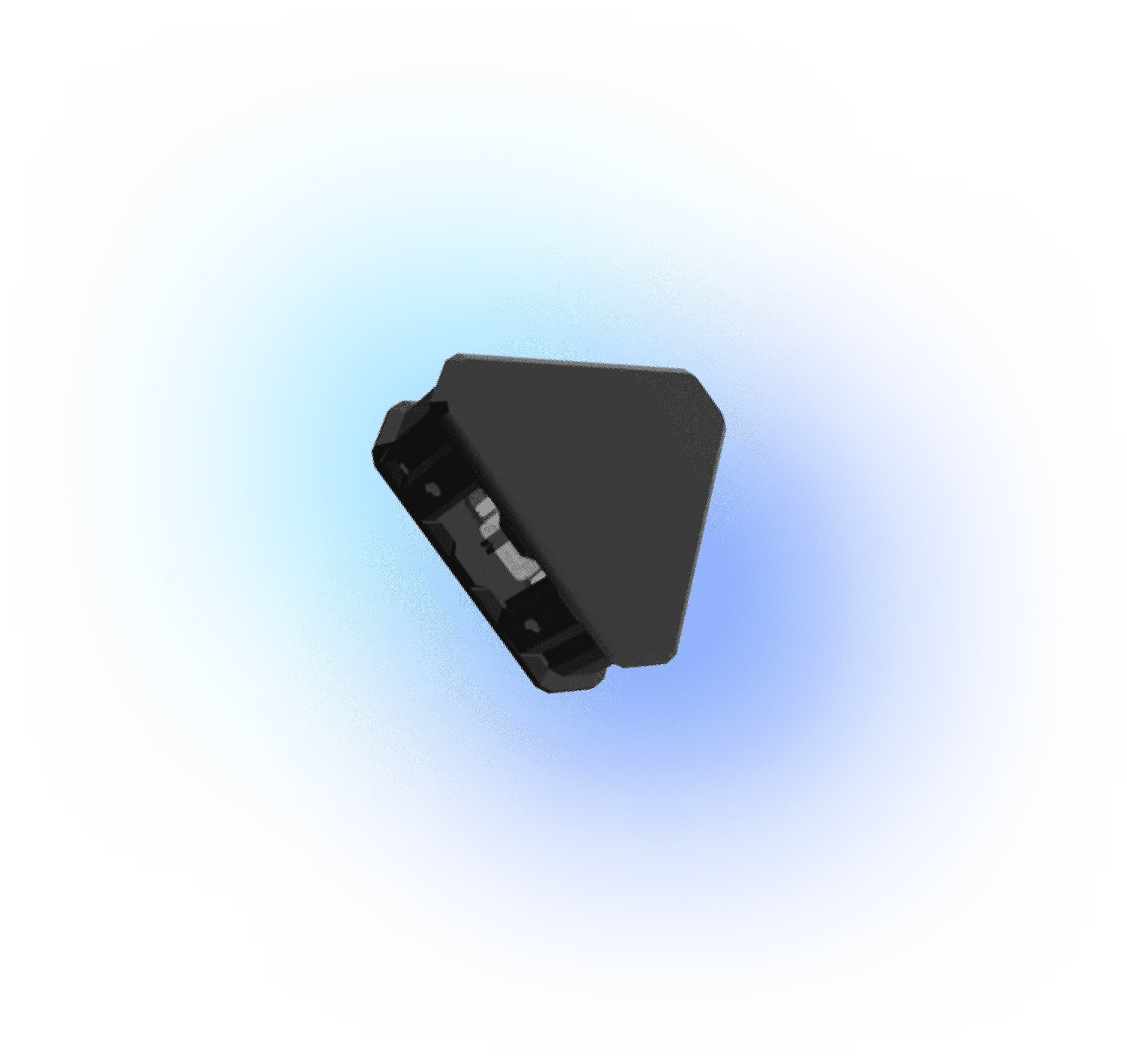
What is Circular reference detected Error in Flutter
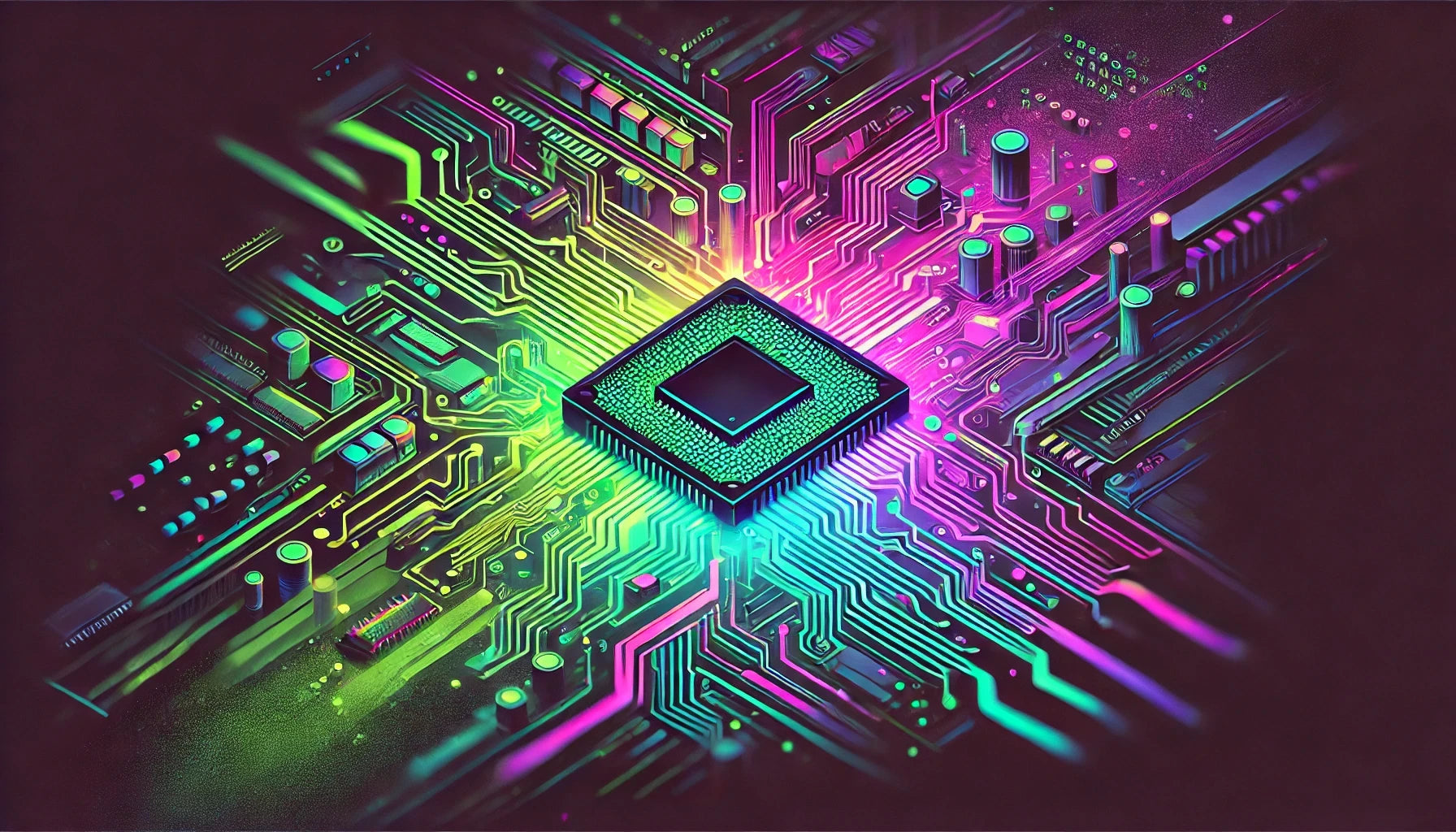
What Causes Circular reference detected in Flutter
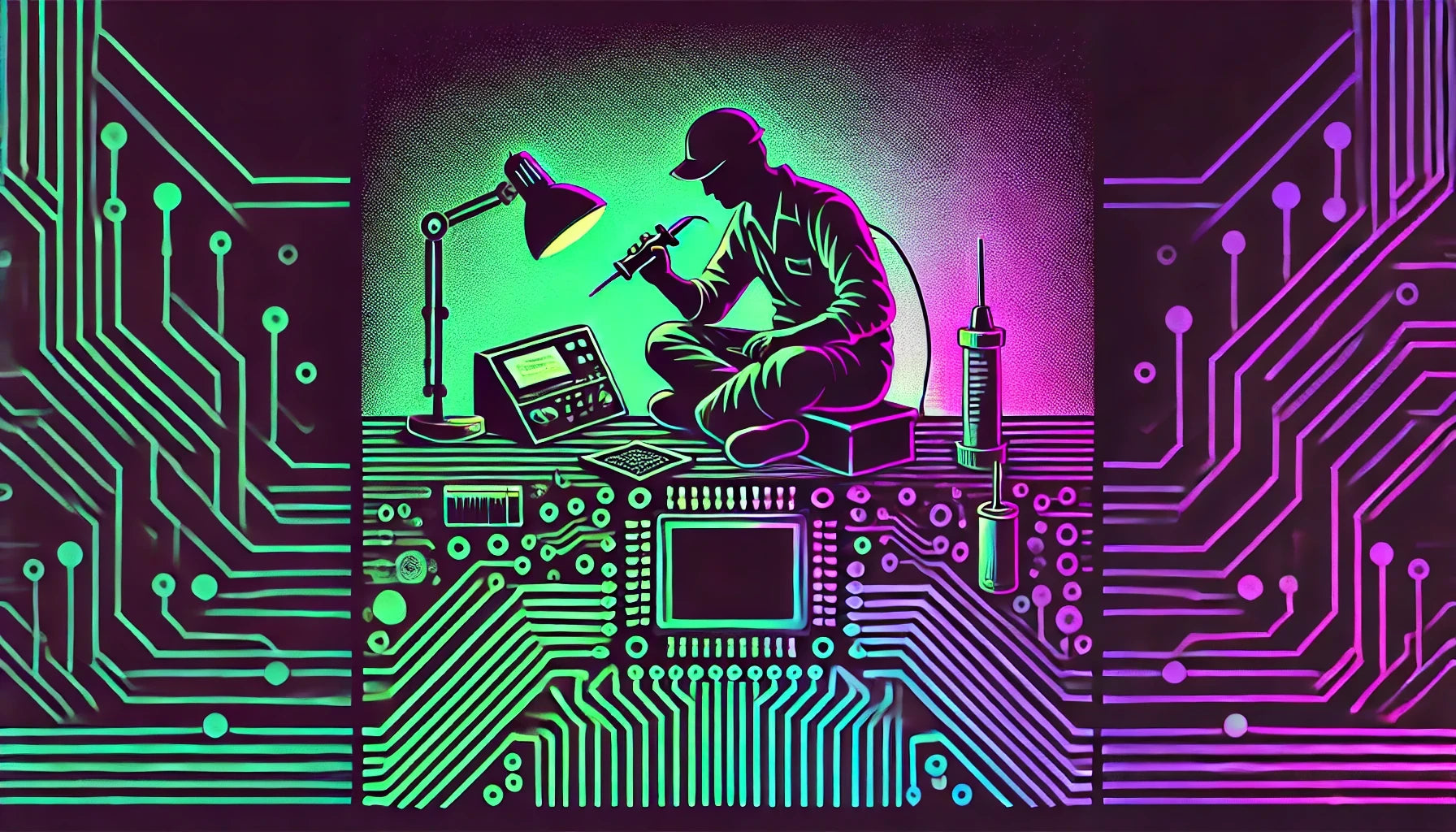
How to Fix Circular reference detected in Flutter
Join the #1 open-source AI wearable community
Build faster and better with 3900+ community members on Omi Discord
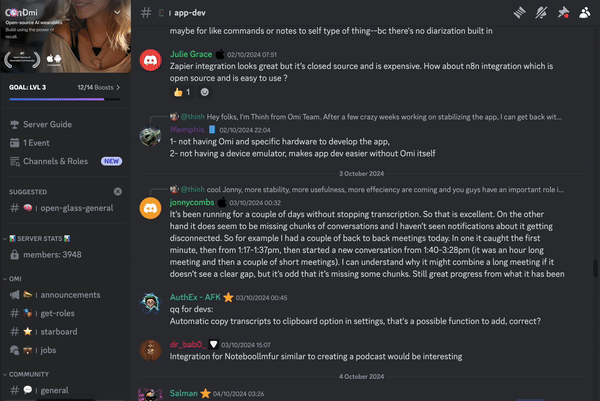
Participate in hackathons to expand the Omi platform and win prizes
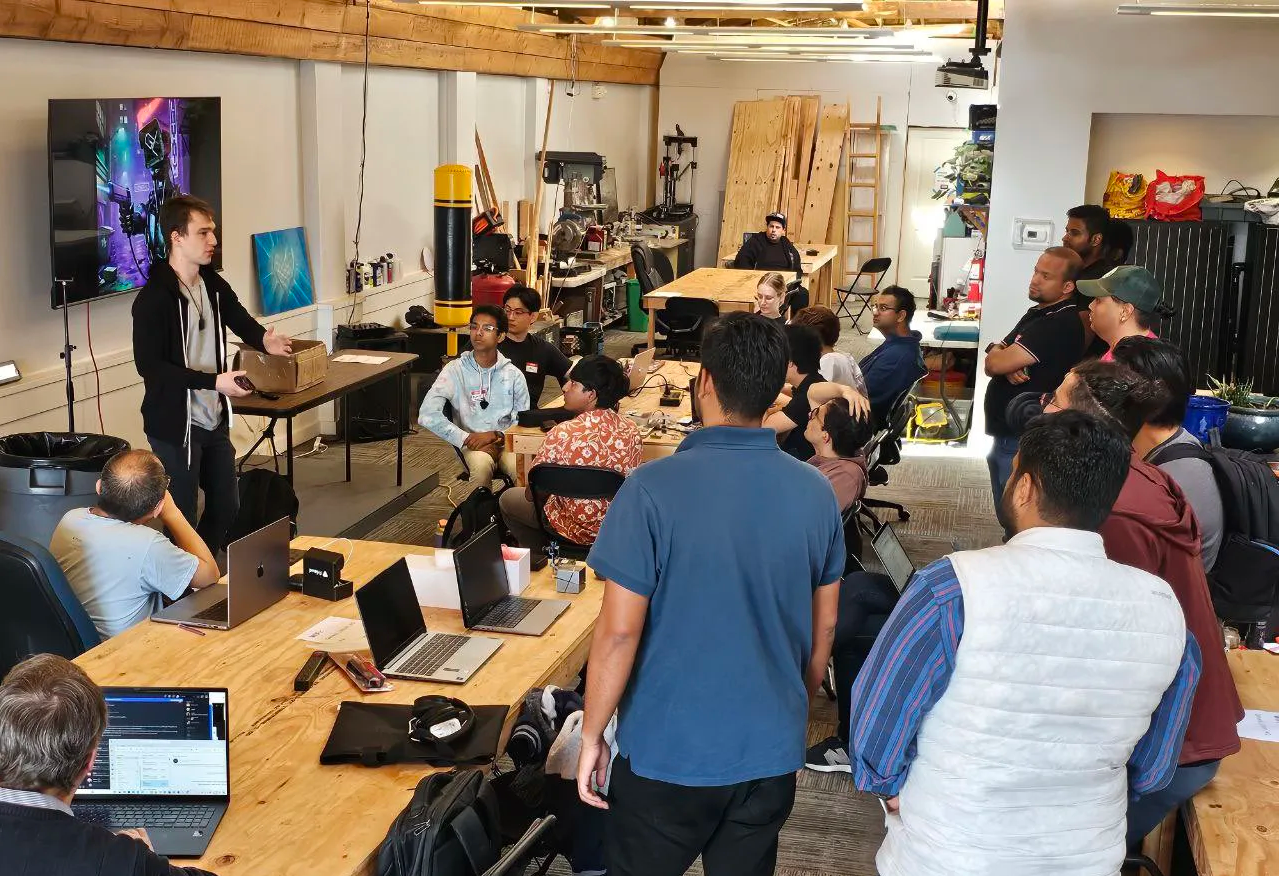
Participate in hackathons to expand the Omi platform and win prizes
Get cash bounties, free Omi devices and priority access by taking part in community activities
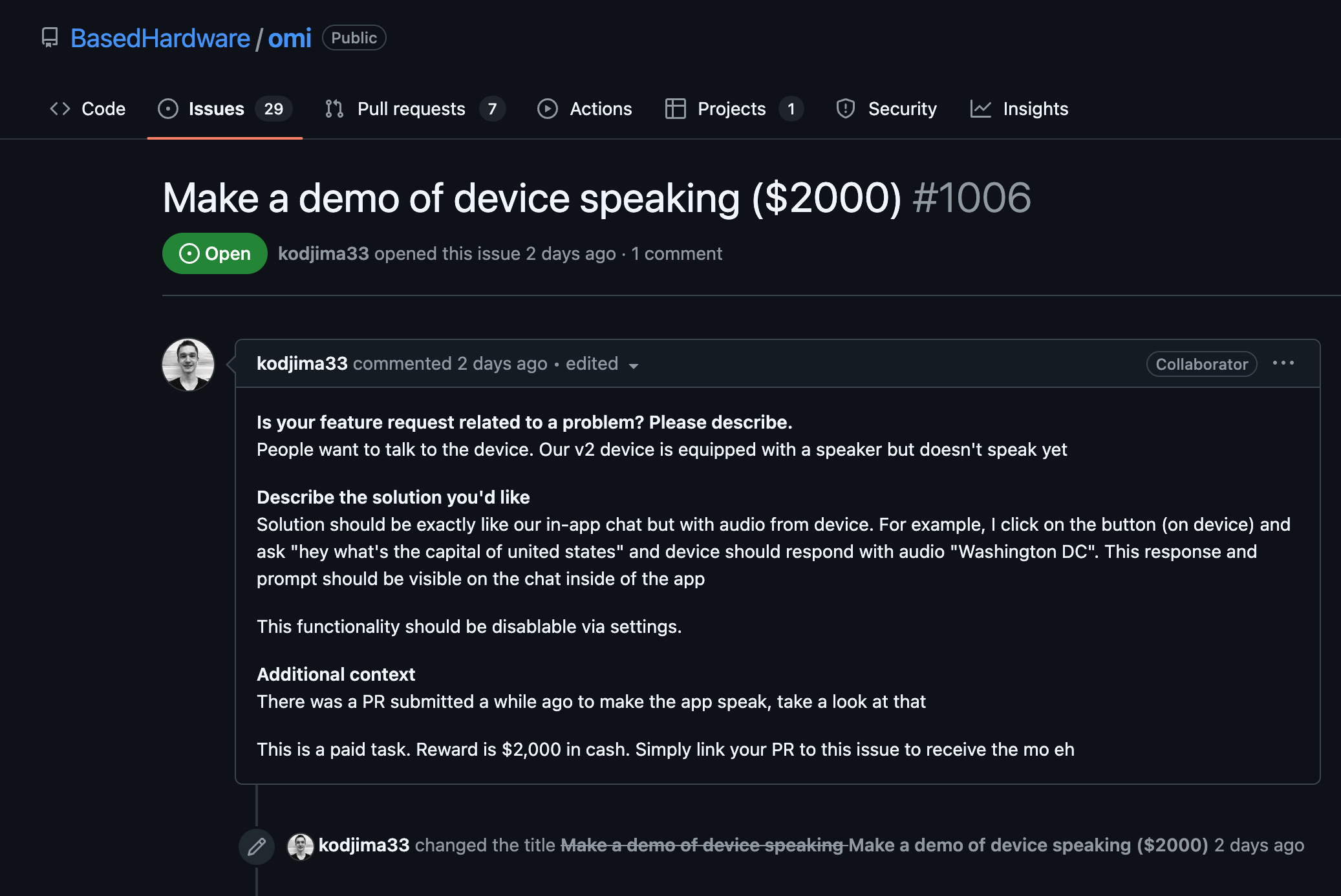
OMI NECKLACE + OMI APP
First & only open-source AI wearable platform











